Node.js MySQL UPDATE
In this post, we will discuss how to delete rows from MySQL XAMPP Server using Node.js
Command to install the mysql package:
Copied
npm install mysql
UPDATE Statement
UPDATE is used to update row/s present in a table. It is important to specify the condition along with this command. Condition can be specified using WHERE Clause.
Steps:Now let's see steps
- First start your XAMPP Server (Both Apache and MySQL).
- Open Notepad or any text-editor and write the Node.js script
- In that script, first we have to load the mysql package using the below syntax var mysql_package = require('mysql');
- Create the connection using the server,username and password.
- Write the sql query that uses UPDATE command
- Now type the following command in your command prompt to run the script. node file_name.js
Copied
var connection_data = mysql_package.createConnection({
host: "localhost",
user: "root",
password: "",
database:"database_name"
});
Copied
connection_data.connect(function(error) {
var sql_query = "UPDATE table_name SET column1=value,column2=value,....
WHERE conditions....";
connection_data.query(sql_query, function (error, result) {
console.log("Row/s updated...");
});
});
Consider the village table with the following records:
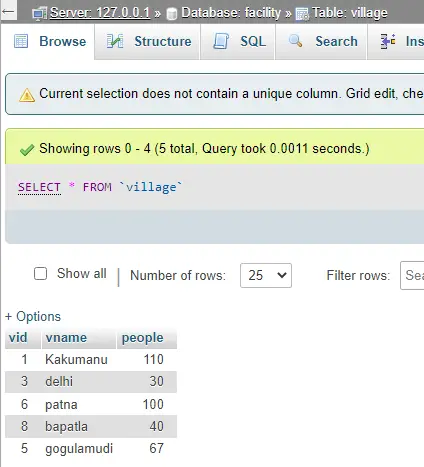
UPDATE Example 1:-
Let's update vname to Hyd in village table where vid is 1.
Copied
// Load the mysql package
var mysql_package = require('mysql');
// Create the connection using the server,username and password.
//In my scenario - server is the localhost,
//username is root,
//password is empty.
//database is facility
var connection_data = mysql_package.createConnection({
host: "localhost",
user: "root",
password: "",
database:"facility"
});
connection_data.connect(function(error) {
// Query to update vname to Hyd if vid=1.
var sql_query = "UPDATE village SET vname = 'Hyd' WHERE vid = 1";
connection_data.query(sql_query, function (error, result) {
console.log(result.affectedRows + " row updated successfully");
});
});
Output:
Copied
1 row updated successfully
Let's check in our XAMPP Server whether the rows updated or not.
UPDATE Example 2:-
Let's update vname to other in village table where people is greater than 50.
Copied
// Load the mysql package
var mysql_package = require('mysql');
// Create the connection using the server,username and password.
//In my scenario - server is the localhost,
//username is root,
//password is empty.
//database is facility
var connection_data = mysql_package.createConnection({
host: "localhost",
user: "root",
password: "",
database:"facility"
});
connection_data.connect(function(error) {
// Query to update vname to other if people> 50.
var sql_query = "UPDATE village SET vname = 'other' WHERE people>50";
connection_data.query(sql_query, function (error, result) {
console.log(result.affectedRows + " rows updated successfully");
});
});
Output:
Copied
3 rows updated successfully
Let's check in our XAMPP Server whether the rows updated or not.
In this post, we seen how to update records using UPDATE Command in XAMPP Server with two Node.js scripts.