Java if else
In this tutorial, we will learn about if, if - else, and else-if ladder statements of java with syntax, flow diagram of each statement and an example of it.
What is an if-else condition:
In the computer programming languages programmers needs to evaluate the conditions based on the evaluated condition result requires to perform the operations. Suppose a student should acquire based marks in order to qualify the test, otherwise student will be dis-qualified, in this condition based on the marks result should be displayed cannot execute same block of statements for different marks.
Then condition should be If marks greater than qualified marks student marked as qualified else student is dis-qualified.
If-else condition in Java
Like many other programming languages Java also supports if-else condition with slight change in the syntax.
Syntax:
if(condition) {
} else {
}
if condition alone can be used (or) using of else block is optional or depends upon the requirement.
Types of if condition:
if and else statements are used to control the execution flow of statements based on one or more conditions and we have the following types of if condition, including else and including with else-if.
- if condition
- if and else condition
- if, else if and else condition.
if condition and its flow chart
if condition prevents or executes its block based on the condition result associated with it.
Syntax:
if(condition) {
// block code executed when condition is true
}
Condition can be anything but the result should boolean value either true or false, multiple condition can be passed by using the logical operators.
A method can be passed as condition to the if statement but the return type of the method should be a boolean type.
Example:
public class IfExample {
public static void main(String[] args) {
int digit = 1000;
if (digit > 100) {
System.out.println("Given number is greater than 100");
}
}
}
Output:
Given number is greater than 100
Above output gets printed after running the example, lets understand the execution control.
- Declared a digit variable with type Integer and value as 1000
- 1000 is greater than 100 condition is true.
- Statements under if block gets executed the print statement.
The following is the flow diagram of the if statement, when its true if block gets executed and false will skip its execution.
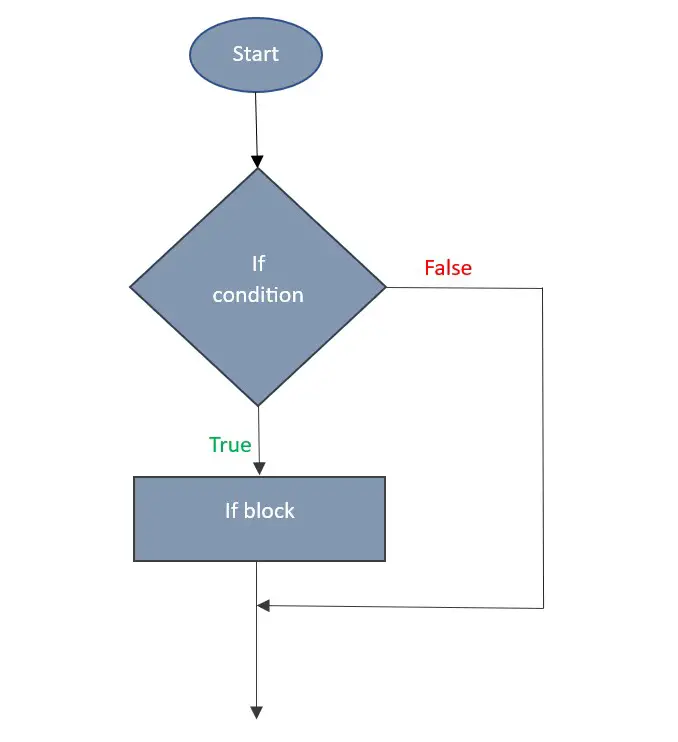
If else condition and its flow chart
if else condition comes handy to execute a block of statements when the condition is not satisfied. Still in the same manner If allows to execute only when condition is satisfied and else block gets executed only when condition is not satisfied.
Syntax:
if(condition) {
// gets executed when condition is true
}
else {
// gets executed when condition is false
}
Example:
public class IfElseExample {
public static void main(String[] args) {
int digit = 10;
if (digit > 100) {
System.out.println("Given number is greater than 100");
}
else {
System.out.println("Given number is less than 100");
}
}
}
Output:
Given number is less than 100
Above line gets printed after executing the example, lets understand how the else condition executed.
- Declared a digit variable with type Integer and value as 10
- 10 is not greater than 100 condition is not satisfied.
- If block statements not executed.
- Condition is not satisfied therefore else block statements are executed and prints the line.
The following is the flow diagram of the if else statement shows flow execution steps.
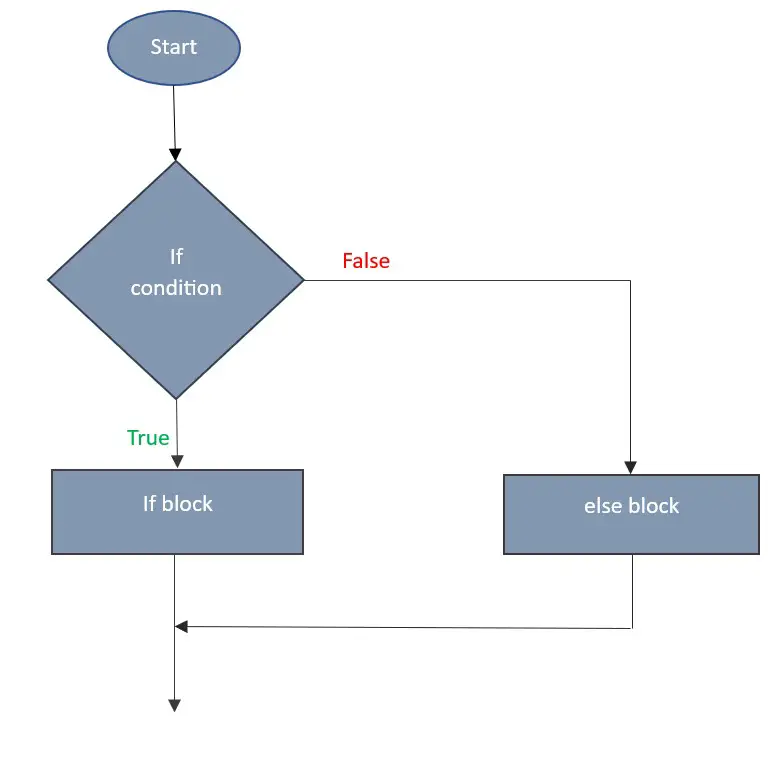
If with else if and else condition including its flow chart
If and else if will allows to perform alternative conditions check and perform alternative actions. Consider we have a job to post grade of students based on marks.
- Student who scored above 90 will be under a grade of A+.
- Student who scored above 75 and below 90 will be under a grade of A.
- Student who scored above 40 and below 75 will be under a grade of A-.
- Student who scored below 40 will be no grade.
Considering above criteria, student grade will decide based on marks and it may vary from student to student in terms of marks scored.
Example:
public class IfElseIfExample {
public static void main(String[] args) {
int stdMarks = 76;
if(stdMarks >= 90) {
System.out.print("Student under Grade A+");
}
else if(stdMarks >= 75) {
System.out.print("Student under Grade A");
}
else if(stdMarks >= 40) {
System.out.print("Student under Grade A-");
}
else {
System.out.print("No grade");
}
}
}
Output:
Student under Grade A
Above line gets printed after executing the example, lets understand how the flow executed.
- Declared the student marks as 76.
- Checks condition of if, whether 76 is greater than or equals to 90.
- Condition is not satisfied, skips execution of if block.
- Checks condition of else if, whether 76 is greater than or equals to 75.
- Condition satisfied, if else block gets executed and remaining condition checks will be skipped.
The following is the flow diagram of if , if else and else condition shows how flow gets executed.
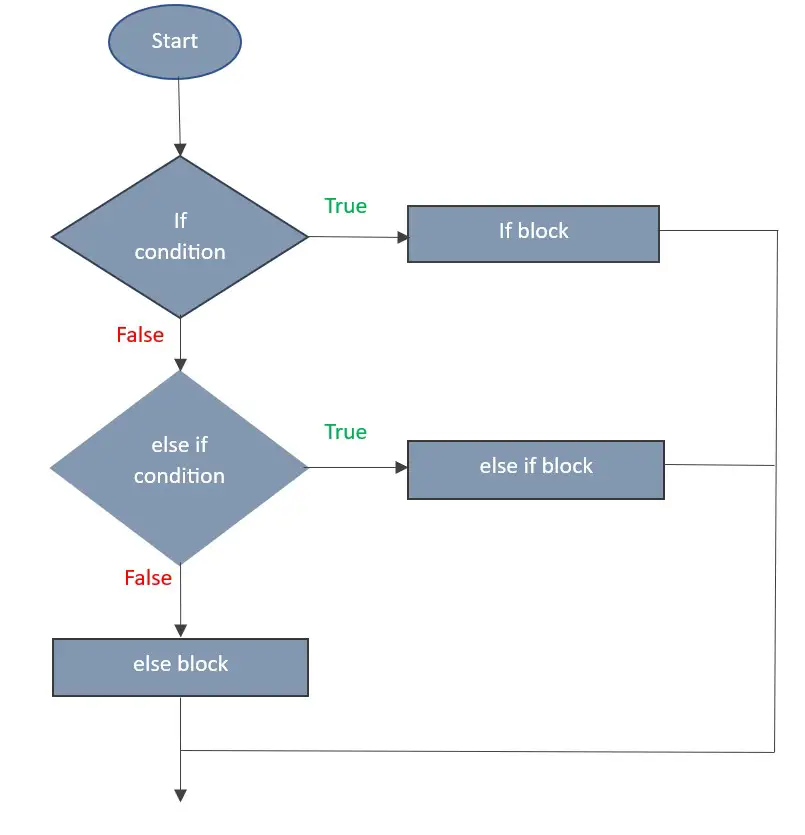
Conclusion:
In this tutorial, we have learned about if, if else and if, else if and else conditions with working example along with the flow diagrams.