Java Thread Life Cycle
In this tutorial, we will learn about what is the life cycle of a Java thread, and explanation of each life cycle with the help of diagram and working example.
Thread Life Cycle
In Java, a thread goes through a different stages or states during its complete execution of a task or set of instructions assigned to it, known as a thread lifecycle. Based on the state of a thread can be determined what exactly its doing, understanding lifecycles of threads is very important for java application developers.
Java thread life cycle consists six states, described following.
- NEW
- RUNNABLE
- BLOCKED
- WAITING
- TIMED_WAITING
- TERMINATED
NEW life cycle state
When we get a thread state with result as NEW, it means thread is created but not started yet.
The following example will demonstrate the thread state which is NEW.
//Created a Class and extends Thread Class
public class ThreadStates extends Thread{
public static void main(String[] args) {
//Initialized ThreadStates Class
ThreadStates threadstate = new ThreadStates();
//Get the thread state, which is not started
System.out.println("State of a Thread : "+ threadstate.getState());
}
}
Output:
State of a Thread : NEW
As per the above example, we created a new thread but its not started hence it returning the state as NEW.
RUNNABLE life cycle state
Thread state which is RUNNABLE means, thread which is currently executing instructions or it may be waiting for the resources of O/S such as CPU or Processor.
The following example will demonstrate the thread state which is RUNNABLE.
//Created a Class and extends Thread Class
public class ThreadStates extends Thread{
public static void main(String[] args) {
//Initialized ThreadStates Class
ThreadStates threadstate = new ThreadStates();
//Starting the Thread
threadstate.start();
//Get the thread state, which is Runnable
System.out.println("State of a Thread : "+ threadstate.getState());
}
public void run() {
// Write a code to be executed by thread
}
}
Output:
State of a Thread : RUNNABLE
As per the above example, we created a new thread and started it hence it returning the state as RUNNABLE.
BLOCKED life cycle state
Thread state which is BLOCKED means, thread which is currently waiting to acquire a monitor lock.
The following example will print the thread state which is BLOCKED.
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class ThreadStates extends Thread {
static Object obj = new Object();
// Initialized ThreadStates Class first thread
static ThreadStates threadstate = new ThreadStates();
// Initialized ThreadStates Class second thread
static ThreadStates threadstate2 = new ThreadStates();
public static void main(String[] args) {
threadstate.setName("Thread First");
// Set the name and start Thread One
threadstate.start();
// Set the name and start Thread two
threadstate2.setName("Thread Second");
threadstate2.start();
}
public void run() {
try {
synchronized (obj) {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
State state = ThreadStates.threadstate2.getState();
System.out.println("Thread Second State :" + state);
String s = this.getName().contains("First") ? br.readLine() : "No Input";
}
} catch (Exception e) {
}
}
}
Output :
Thread Second State :BLOCKED
Thread Second State :RUNNABLE
Note: While running above example, press enter button after you see output of first line otherwise it will wait forever for your input.
Explanation of above output, second thread will be in blocked state until you press a Enter after that it will goes to RUNNABLE state.
WAITING life cycle state
Thread state which is WAITING means, thread which is executed wait
()
method and waiting for other thread to invoke notify()
or notify()
.
This state can also occur if a current thread calls a join()
method.
public synchronized void resourceMethod() throws Exception {
wait();
}
TIMED WAITING life cycle state
Thread state which is TIMED WAITING means, thread which is executed wait
or sleep
method with timeout parameter in milli seconds.
public synchronized void resourceMethod() throws Exception {
sleep(100);
}
TERMINATED state
After completed its execution of a thread will give the result state as TERMINATED, it means thread completed its execution of set of instructions.
The following diagram shows all the life cycles of threads, bi-directional arrows can get back to the same state but unidirectional arrows will not come to same state.
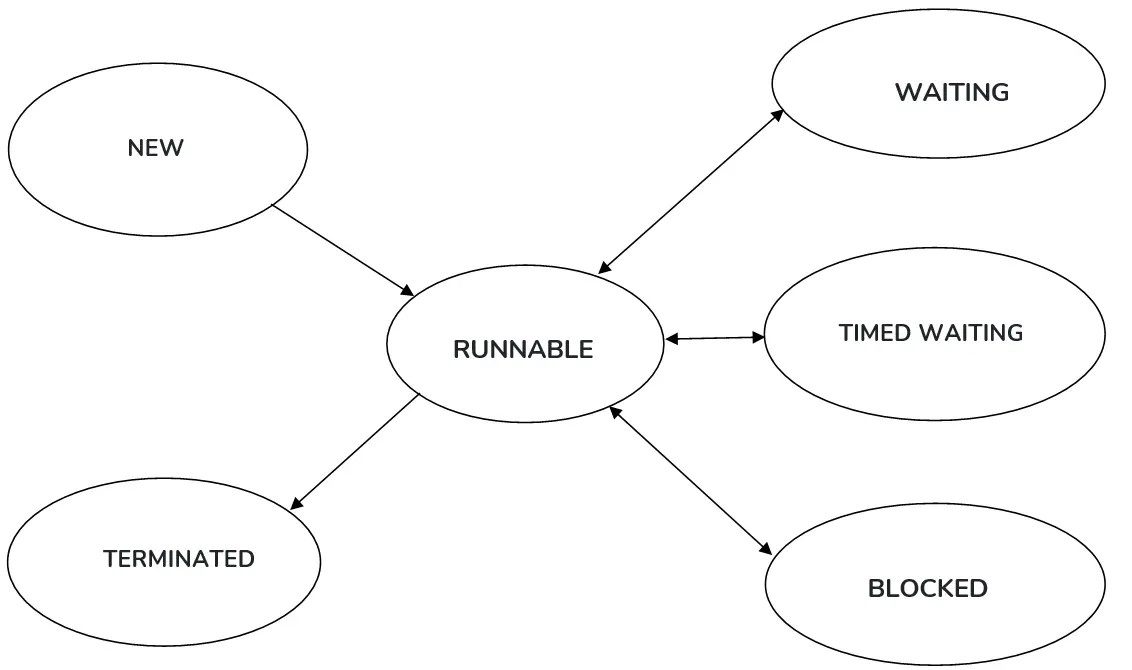
Conclusion:
In this tutorial, we have covered life cycle of Java threads and explanation of each with the example and with the help of diagram also.