Python Method Resolution
In the multiple inheritance scenario, any specified attribute or method is searched first in the current class. If not found, the search continues into parent classes in depth-first, left to right fashion without searching the same class twice. Searching in this way is called Method Resolution Order (MRO).
Method Resolution principles
- The first principle is to search for the sub class before going for its base classes. Thus if class B is inherited from A, it will search B first and then goes to A.
- The second principle is that when a class is inherited from several classes, it searches in the order from left to right in the base classes. For example, if class C is inherited from A and B as class C(A,B), then first it will search in A and then in B.
- The third principle is that it will not visit any class more than once. That means a class in the inheritance hierarchy is traversed only once exactly.
Understanding MRO gives us clear idea regarding which classes are executed and in which sequence. We can easily estimate the output when several base classes are involved.
Method Resolution Hierarchy
The below image shows the hierarchy flow of method resolution.
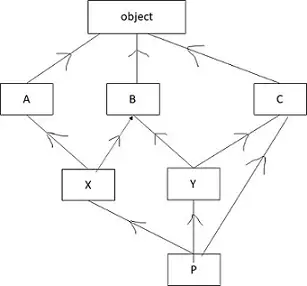
Method Resolution Example :
Copied
class A(object):
def method(self):
print('A class method')
super().method()
class B(object):
def method(self):
print('B class method')
super().method()
class C(object):
def method(self):
print('C class method')
class X(A, B):
def method(self):
print('X class method')
super().method()
class Y(B, C):
def method(self):
print('Y class method')
super().method()
class P(X,Y,C):
def method(self):
print('P class method')
super().method()
p = P()
p.method()