Python OOPS Features
There are five important features related to Object Oriented Programming System.
They are as follows
- Classes and objects
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
Classes and Objects
The entire OOPS methodology has been derived from a single root concept called 'object'.
An object is anything that really exists in the world and can be distinguished from others.
This definition specifies that everything in this world is an object. For example, a table, a ball, a car, a dog, a person, etc. will come under objects.
A class is a user defined blueprint or prototype from which objects are created.
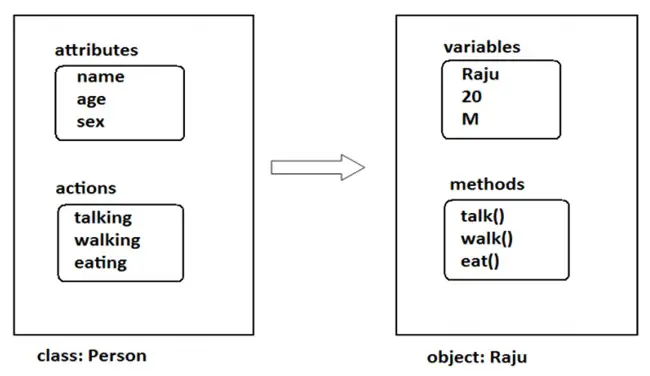
Creation of a Class and Objects in Python
Let's create a class with the name Person for which Raju and Sita are objects.
A class is created by using the keyword, 'class '.
A class describes the attributes and actions performed by its objects.
So, we write the attributes (variables) and actions (functions) in the class as:
class Person:
name = 'Raju'
age = 20
#actions means functions
def talk(cls):
print(cls.name)
print(cls.age)
Person class has two variables and one function. The function that is written in the class is called method . When we want to use this class, we should create an object to the class as:
p1 = Person()
Here, p1 is an object of Person class. Object represents memory to store the actual data. The memory needed to create p1 object is provided by PVM.
Observe the function (or method) in the class:
def talk(cls):
Here, 'cls' represents a default parameter that indicates the class. So, cls.name refers to class variable 'Raju'. We can call the talk() method to display Raju's details as:
p1.talk()
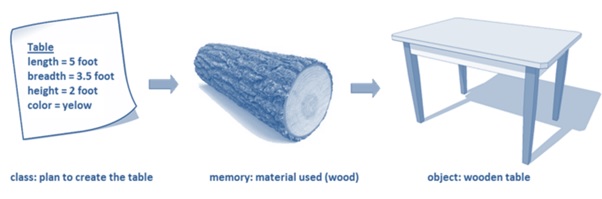
Encapsulation
Encapsulation is a mechanism where the data (variables) and the code (methods) that act on the data will bind together. For example, if we take a class, we write the variables and methods inside the class. Thus, class is binding them together. So class is an example for encapsulation.
For example, we can write a Student class with 'id' and 'name' as attributes along with the display() method that displays this data. This Student class becomes an example for encapsulation.
class Student:
def __init__(self):
self.id = 10
self.name = 'Raju'
#display students details
def display(self):
print(self.id)
print(self.name)
Abstraction
There may be a lot of data, a class contains and the user does not need the entire data. The user requires only some part of the available data. In this case, we can hide the unnecessary data from the user and expose only that data that is of interest to the user. This is called abstraction.
Abstraction in Python
In languages like Java, we have keywords like private, protected and public to implement various levels of abstraction. These keywords are called access specifiers. In Python, such words are not available. Everything written in the class will come under public. That means everything written in the class is available outside the class to other people. Suppose, we do not want to make a variable available outside the class or to other members inside the class, we can write the variable with two double scores before it as: __var. This is like a private variable in Python . In the following example, 'y' is a private variable since it is written as: __y.
class Myclass:
#this is constructor.
def __init__(self):
self.__y = 3 #this is private variable
Now, it is not possible to access the variable from within the class or out of the class as:
m = Myclass()
print(m.y) #error
The preceding print() statement displays error message as: AttributeError: 'Myclass' object has no attribute 'y'. Even though, we cannot access the private variable in this way, it is possible to access it in the format:
instancename._Classname__var.
That means we are using Classname differently to access the private variable. This is called name mangling. In name mangling, we have to use one underscore before the classname and two underscores after the classname. Like this, using the names differently to access the private variables is called name mangling.
Inheritance
Creating new classes from existing classes, so that the new classes will acquire all the features of the existing classes is called Inheritance. A good example for Inheritance in nature is parents producing the children and children inheriting the qualities of the parents.
Polymorphism
The word 'Polymorphism' came from two Greek words 'poly' meaning 'many' and 'morphos' meaning 'forms'. Thus, polymorphism represents the ability to assume several different forms. In programming, if an object or method is exhibiting different behavior in different contexts, it is called polymorphic nature. Polymorphism provides flexibility in writing programs in such a way that the programmer uses same method call to perform different operations depending on the requirement.
When a function can perform different tasks, we can say that it is exhibiting polymorphism
#a function that exhibits polymorphism
def add(a, b):
print(a+b)
#call add() and pass two integers
add(5, 10) #displays 15
#call add() and pass two strings
add("Core", "Python") #displays CorePython
Points to Remember
- Procedure oriented approach is the methodology where programming is done using procedures and functions. This is followed by languages like C, Pascal and FORTRAN.
- Object oriented approach is the methodology where programming is done using classes and objects. This is followed in the languages like C++, Java and Python.
- Python programmers can write programs using procedure oriented approach (like C) or object oriented approach (like Java) depending on their requirements.
- An object is anything that really exists in the world and can be distinguished from others.
- Every object has some behavior that is characterized by attributes and actions. Attributes are represented by variables and actions are performed by methods. So an object contains variables and methods.
- A function written inside a class is called method.
- A class is a model or blueprint for creating objects. A class also contains variables and methods.
- Objects are created from a class.
- An object does not exist without a class; however, a class can exist without any object.
- Encapsulation is a mechanism where the data (variables) and the code (methods) that act on the data will bind together.
- Class is an example for encapsulation since it contains data and code.
- Hiding unnecessary data and code from the user is called abstraction.
- To hide variables or methods, we should declare them as private members. This is done by writing two underscores before the names of the variable or method.
- Private members can be accessed using name mangling where the class name is used with single underscore before it and two underscores after it in the form of: instancename. _Classname__variable or instancename. _Classname__method().
- Creating new classes from existing classes, so that new classes will acquire all the features of the existing classes is called Inheritance.
The programming languages which follow all the five features of OOPS are called object oriented programming languages. For example, C++, Java and >Python will come into this category.